Snippets
参考:https://medium.com/better-programming/20-python-snippets-you-should-learn-today-8328e26ff124
序列定长分割
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| In [16]: from six.moves import zip_longest
In [17]: for i in zip_longest(*[iter([1,2,3,4,5])]*2, fillvalue=0): ...: print(i) ...: (1, 2) (3, 4) (5, 0)
In [18]: for i in zip_longest(*[iter('abcdefg')]*2, fillvalue='*'): ...: print(i) ...: ('a', 'b') ('c', 'd') ('e', 'f') ('g', '*')
|
字符串逆转
1 2 3 4
| In [1]: my_string = "ABCDE"
In [2]: my_string[::-1] Out[2]: 'EDCBA'
|
标题类(首字母大写)
1 2 3 4
| In [3]: my_string = "aaa bbb ccc ddd"
In [4]: my_string.title() Out[4]: 'Aaa Bbb Ccc Ddd'
|
n次序列
1 2 3 4 5 6 7 8 9
| In [6]: my_string = "abcd"
In [7]: my_string*3 Out[7]: 'abcdabcdabcd'
In [8]: my_list = [1,2,3,4]
In [9]: my_list*3 Out[9]: [1, 2, 3, 4, 1, 2, 3, 4, 1, 2, 3, 4]
|
列表解析
1 2 3 4 5 6 7 8 9 10 11 12
| In [8]: my_list=[1,2,3,4,5]
In [9]: [x for x in my_list if x%2!=0] Out[9]: [1, 3, 5]
In [10]: my_list = [1,2,3,4]
In [11]: new_list = [2*x for x in my_list]
In [12]: new_list Out[12]: [2, 4, 6, 8]
|
交换值
1 2 3 4 5 6
| In [15]: a,b=1,2
In [16]: a,b = b,a
In [17]: a Out[17]: 2
|
连接字符串
1 2 3 4
| In [18]: list_of_strings = ['My', 'name', 'is', 'Chaitanya', 'Baweja']
In [19]: ','.join(list_of_strings) Out[19]: 'My,name,is,Chaitanya,Baweja'
|
列表计数
1 2 3 4 5 6 7 8 9 10 11
| In [23]: from collections import Counter
In [24]: my_list = ['a','a','b','b','b','c','d','d','d','d','d']
In [25]: count=Counter(my_list)
In [26]: count Out[26]: Counter({'a': 2, 'b': 3, 'c': 1, 'd': 5})
In [27]: count.most_common(1) Out[27]: [('d', 5)]
|
合并字典
1 2 3 4 5 6 7 8
| In [28]: dict_1 = {'apple': 9, 'banana': 6}
In [29]: dict_2 = {'banana': 4, 'orange': 8}
In [30]: combined_dict = {**dict_1, **dict_2}
In [31]: combined_dict Out[31]: {'apple': 9, 'banana': 4, 'orange': 8}
|
列表扁平化
1 2 3 4 5 6
| In [1]: from iteration_utilities import deepflatten
In [2]: l = [[1,2,3],[4,[5],[6,7]],[8,[9,[10]]]]
In [3]: print(list(deepflatten(l, depth=3))) [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
|
列表采样
1 2 3 4 5 6 7 8
| In [4]: import random
In [5]: my_list = ['a', 'b', 'c', 'd', 'e']
In [6]: num_samples = 2
In [7]: random.sample(my_list,num_samples) Out[7]: ['c', 'a']
|
对象内存
1 2 3 4 5 6
| In [5]: import sys
In [6]: a=10
In [7]: sys.getsizeof(a) Out[7]: 28
|
常见问题
集合运算
注:集合不支持索引访问
操作符号列表
含义 | python |
---|
差集 | -/difference() |
交集 | &/intersection |
并集 | |/union |
不等于 | != |
等于 | == |
属于 | in |
不属于 | not in |
init.py 作用
https://www.jianshu.com/p/73f7fbf75183
__init__.py
该文件的作用就是相当于把自身整个文件夹当作一个包来管理,每当有外部import的时候,就会自动执行里面的函数。
二维list赋值问题
参考: (https://blog.csdn.net/zzc15806/article/details/82629406)(https://blog.csdn.net/zzc15806/article/details/82629406)
问题:以 arr = [[0]*N]*N
形式初始化时,对二维数组对某个元素赋值时会发现整列都会被赋值
原因:因为 [[0]*N]*N
表示的是N个指向 [0]*N
这个列表的引用,所以当你修改某一个值时,整个列表都会被改变。
解决:以arr=[[0]*N for _ in range(N)]
赋值。
联系作者
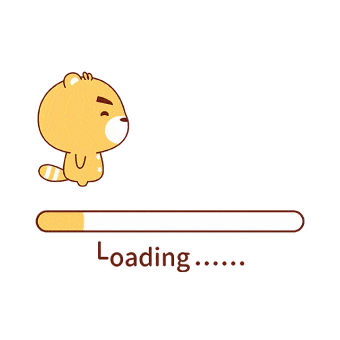